This prototype will follow a different genre to the previous two, being a Top Down Shooter. Once again I’m sticking with the theme of my medieval fantasy as I feel working with ideas that you are interested in can be better for inspiration and motivation. In this game, you will be a lone soldier having to try and hold off an army of enemy soldiers by using a crossbow; somewhat similar to the concept of Fortress Fighter but instead they come from all directions and you can move around the entire map, making the challenge a little more dire to keep the player focused.
Early Stages
The very beginning is akin to that of the previous projects, with it being a 2D Core game I loaded up a fresh Unity project and got ready to prepare my main canvas. However this time I went about things slightly differently, by creating a second scene from the get go which will serve as a main menu; the first time I’ve ever incorporated a mechanic like this into any game. The way I went about this is by going into the scenes folder within Unity and creating a second one labelled ‘MainMenu’ and renaming the primary scene to ‘MainGame’ so I can easily tell one from the other.
The tutorial then taught me how to add and configure particle effects into your game, I went along with the basics before going off on my own a little bit and instead of going with the ‘Stars’ we were being taught to implement I decided to go for flames to use for my main menu rather than my actual game. The reason for this is that I wanted to really represent the aspect of war, linking back to the theme I’m going for. An example of the scene folder and the flames I created are shown below (They are animated, but this is a still image):
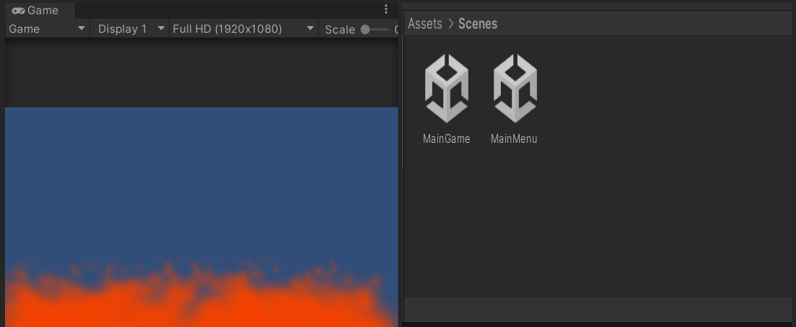
Aswell as these minor additions I then went on to start building what will be my player sprite, for now only using Unity’s own shape game objects until my assets are completed as their implementation can come last as long as I have the skeleton laid out properly. Instead of just one gameobject, this time I required three. These being the player sprite, the weapon atop it, and the barrel of the weapon which I’ve dubbed as ‘Crossbow’ as this will be the weapon that will move with the player and shoot on demand. Shown below is both the sprite on the canvas and the hierarchy representing the layer I’ve done atop the initial player sprite:
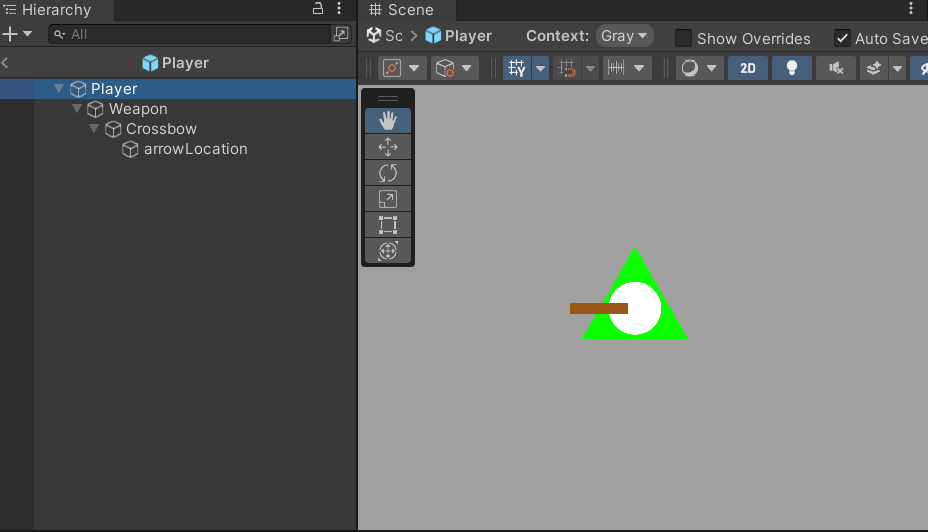
For my next step, using the player sprite that I’ve just put together it was about time that I worked on being able to control it and make it move around the screen using the arrow key inputs just like any other basic game. This time around I also managed to implement boundaries for the canvas that weren’t just physical and unseen walls on the sides of the screen unlike FortressFighter. The way I did this was by hard coding limitations on the player that set maximum boundaries on the edge of the screen to avoid them straying off into the unknown. Labelled code of how I achieved this is shown below:
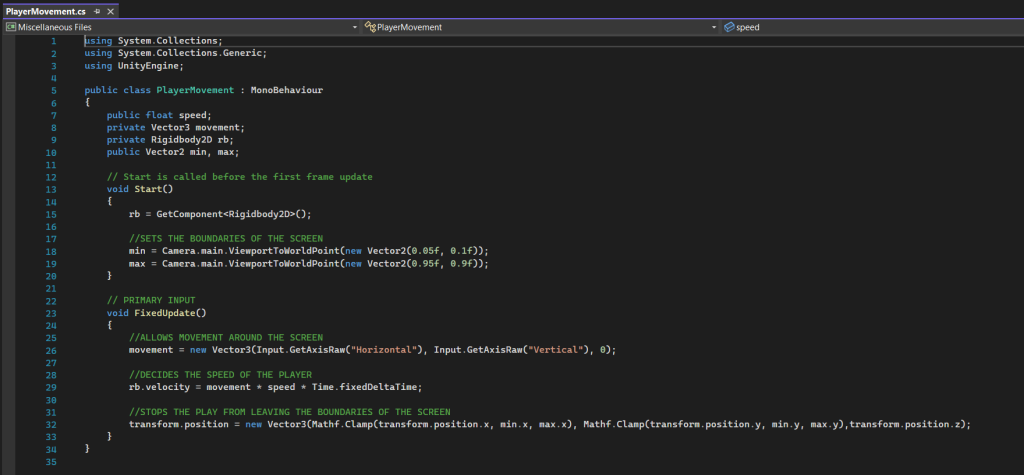
After nailing down the key movement points of the player, now it was time to use some of the parts I’d built with the player game object. This being the weapon, in which it must turn to face the player’s cursor in order to aim and fire. I started this off by first making a crosshair of my own within photoshop, nothing out of the ordinary it’s just a simple combination of two rectangles to form a cross which will server as a reticule for the player to aim at their enemies. This however was only to sit aside until the time came that it was integrating into the aiming system. As for the aiming system it was a rather straight forward approach as it only required a few lines of code, which are shown below:
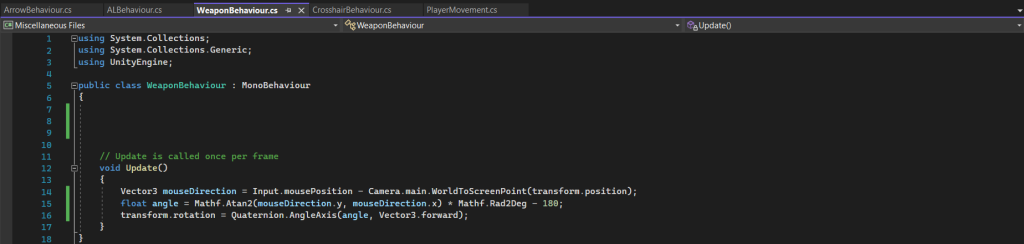
Now that the weapon direction was focused on the cursor, this is where the crosshair came in. Again, a very straight forward script that made it so the cursor was no longer visible on the screen and instead replace with the reticule I had created and followed the location of the mouse on the screen. The lines of code are shown below:
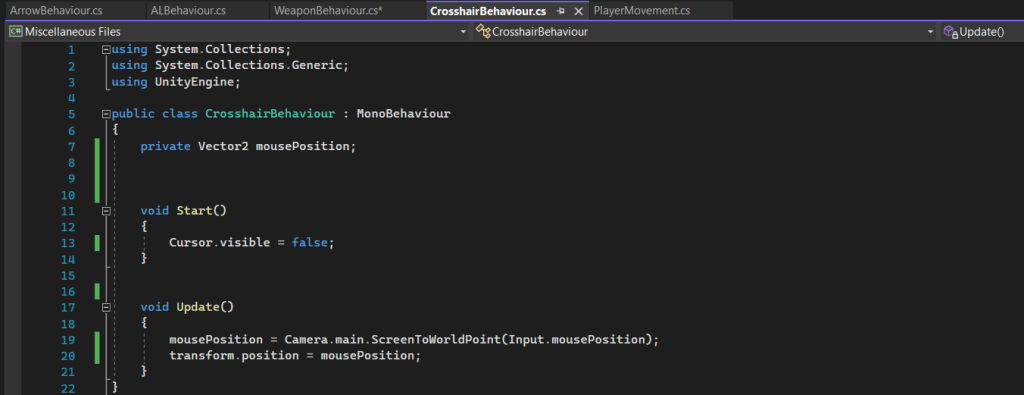
With everything prepared to be able to aim an arrow in a desired location, it was about time I actually created said arrow. Therefore I merely used a shape provided in the basic Unity assets which was a circle and applied a RigidBody 2D aswell as a 2D Circle Collider, not forgetting to change the gravity setting to zero on the RigidBody component as it will end up falling off of the screen. A example of my projectile is shown below alongside the components I’ve configured upon it:
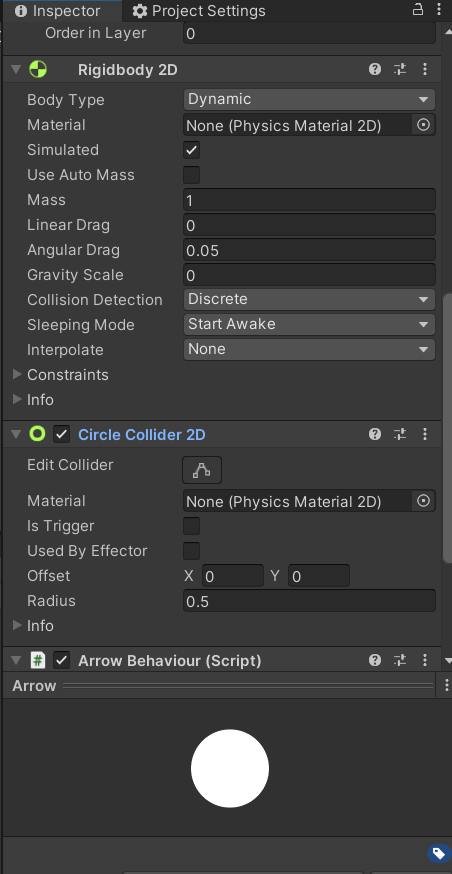
With the creation of my new arrow asset and the foundations of how it will function already set, there was only one last script I needed to write; this being where it travelled and how it travelled. Additionally I also added a function that destroyed the gameobject exactly 1.5 seconds after it launches to avoid the clone clutter and the eventual performance effect that would have. The labelled script is shown below:
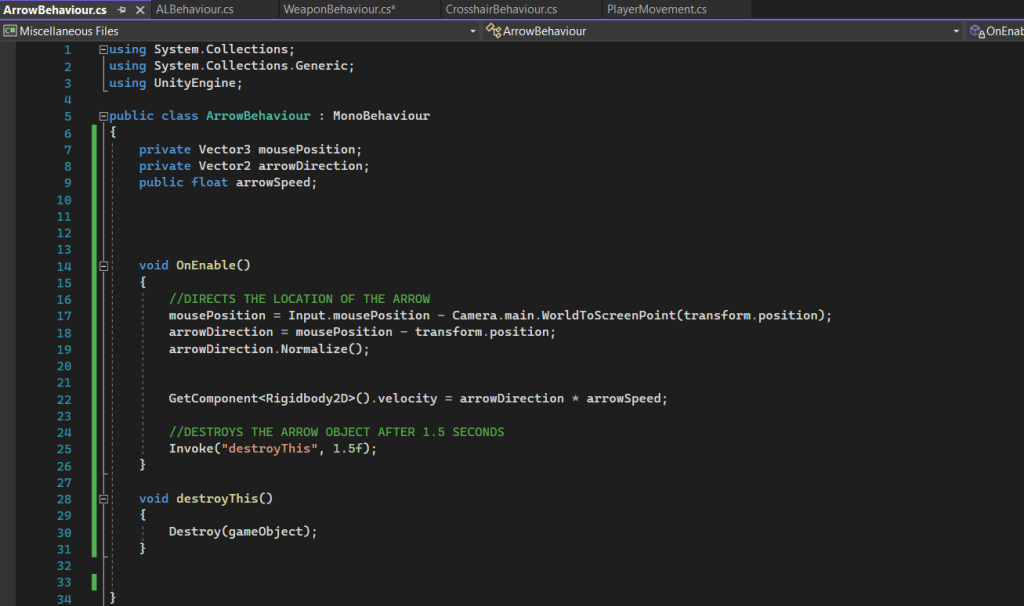
Each of these steps lead to a satisfying result, with the player now being able to move around the canvas and shoot according to where the location of the cursor is. Nonetheless, the most challenging part of the prototype came into play; which was the enemies. Despite the enemies I put in my last game, the complexity of the enemies in this game is far more advanced and beyond a single script or two. This time around it required several scripts in order for the enemies to perform correctly, they go as follows.
The first script was based entirely around when, where and how many enemies spawn when the game begins. To start with, an enemy will spawn around one of the four edges of the map, following this there’ll be a two second time before the next spawns and the timer slowly decreases over time meaning that more enemies begin to spawn. In addition to this there will be three different enemy types that will be covered in one of the next scripts. Labelled examples of the code are shown below (All three screenshots are from the same Script):
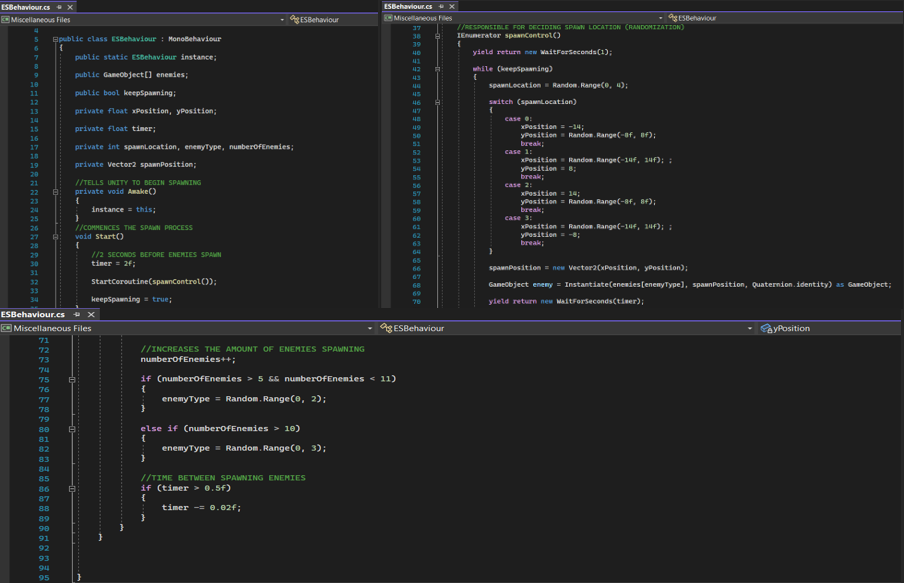
Since the spawn locations are now in place, I could now focus on how the enemies will travel. In this case I’d like the enemies to lock on and directly travel straight towards the player and so this next script is purely based around how fast they’d travel and their primary and only target. Labelled example of the code written is shown below:
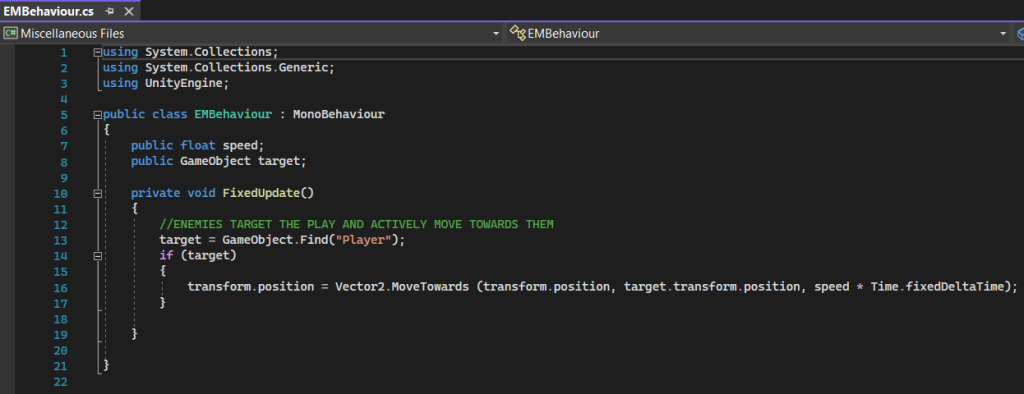
After this was implemented the enemies then aimed straight for the player and moved right for them, however I noticed they all moved the same speed. To amend this I made it so they all moved at various speeds using the public class I created within Unity, for example the first enemies are the slowest, the second enemies faster and the third enemies the fastest. The reason for this is so there’s a sense of thought going into each target, for example the player will want to prioritise the faster enemies as they’re more likely to reach and destroy them. Not to forget that the player is now capable of using the finished firing system to use their projectiles to destroy the enemies that pursue them, meaning that the main mechanics of the game are now complete. A labelled example of the code is shown below:
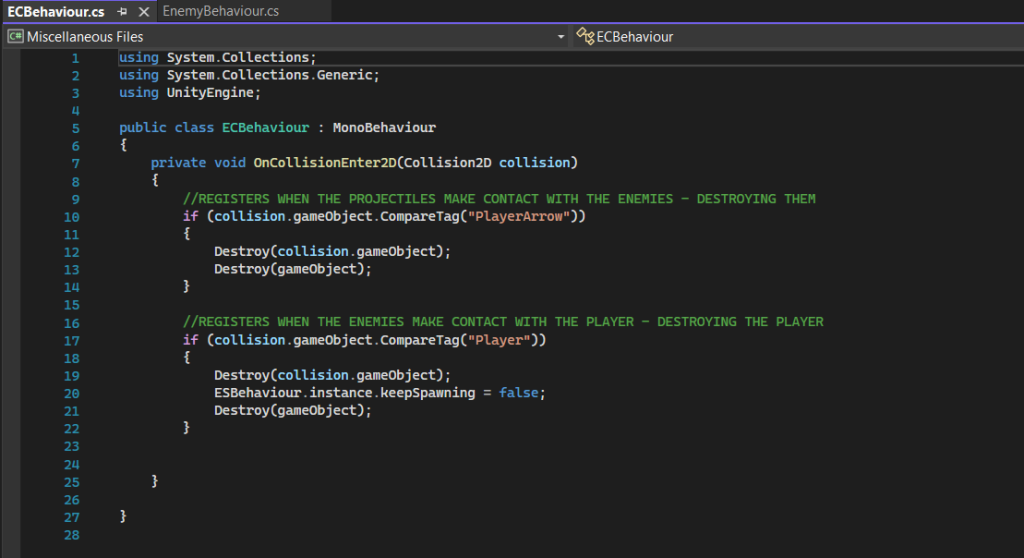
Another minor addition leads us into my next venture; the scoring system which began with creating a public class known as ‘Score’ within a brand new script. This gave me the opportunity to give each different enemy their own score. The code in which is shown below:
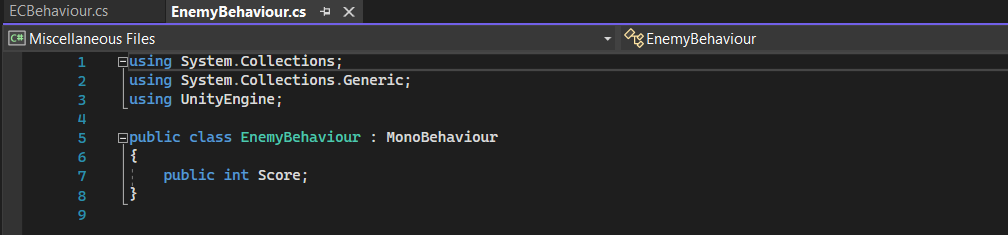
Building off of the basis I laid out in the EnemyBehaviour scripts, I went into Unity and placed a UI Canvas onto my project and then added a Text-TextMeshPro as a child object, dragging it to the corner of the UI Canvas and changing the colour of the text aswell as the outline to let it stick out a little bit. An example is shown below:
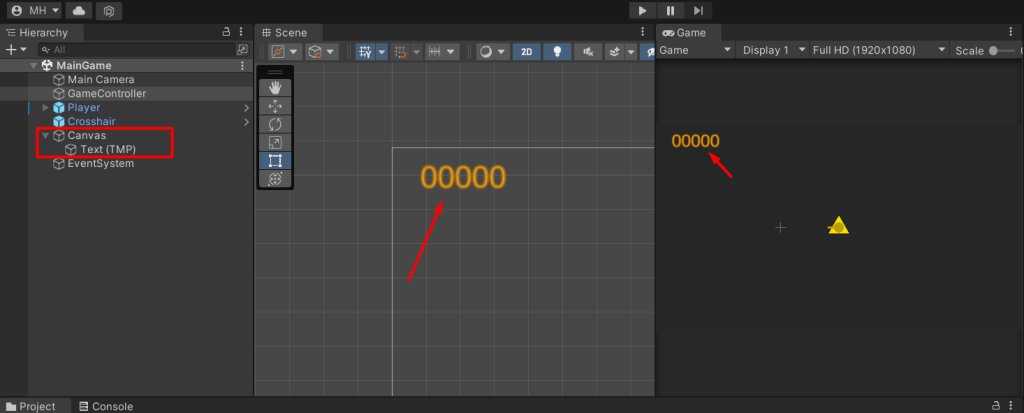
As for the coding side of things, I created a script that contained an integer purely designed to keep track of accumulated score as well as where to present it to the play. However, I soon had to go back to my EnemyCollision script in order to tell Unity when to actually register that score has been achieved. The score earned from each enemy is decided by how fast they are, adding a level of difficulty and reward at the same time.
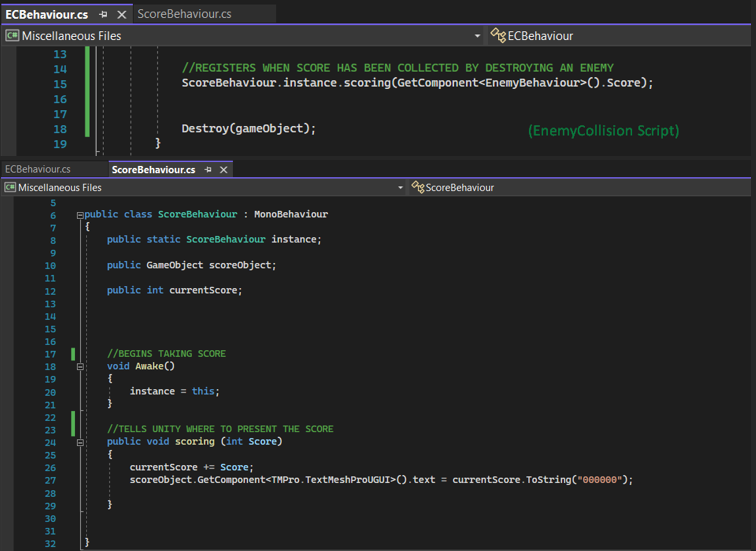
This part of the project is where I had the most fun, upon death enemies with explode into a visual particle effect to make it a little bit more exciting. In addition to this the colour of said explosion will be according to their own sprite’s colour, for example if you destroyed the red enemy their explosion would be red and so on. The way I implemented this was yet again through the EnemyCollision script which registered that when an enemy is destroyed they will explode. The particle effect itself required a lot of experimenting with all the different configurations such as size of lifetime which decided how big the explosion started and how small it would end or the emission sector which allowed me to decide the amount of particles that are displayed at a time. The labelled code is shown below:

The only thing the game needs now is an end when the player eventually loses. When this happens, a screen will appear displaying text telling them the game is over and they can click to try again. Instead of writing out lines of code to do this I instead place a button that spread across the whole scene but is invisible entirely apart from its text in the center of the screen. I used the Game Controller for this and created yet another script that’ll also allow the player to quit the game by pressing ‘Esc’ as well as a timer of one second after the player dies before displaying the game over screen. Lastly I then wrote out a function that reloads the scene when the prompt is clicked, restarting the game. However I then used my knowledge myself of the code from the last step to create an explosion when the player dies too do avoid an abrupt end. The edited code aswell as the fresh script is shown and labelled below:
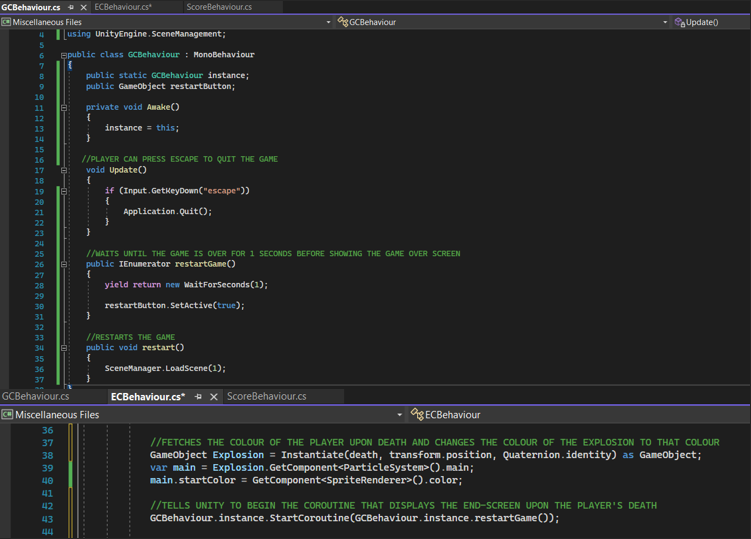
Finally as the prototype comes to a close, the very start comes as the very end. The main menu that players will start the game from needs to have a button that allows them to begin the game, this stage was very simple as it required very few lines of code and little effort to place a button and then bits of text such as the title, button text and instructions that tell the player how to play. Shown below is the code needed and a screenshot of the main menu:
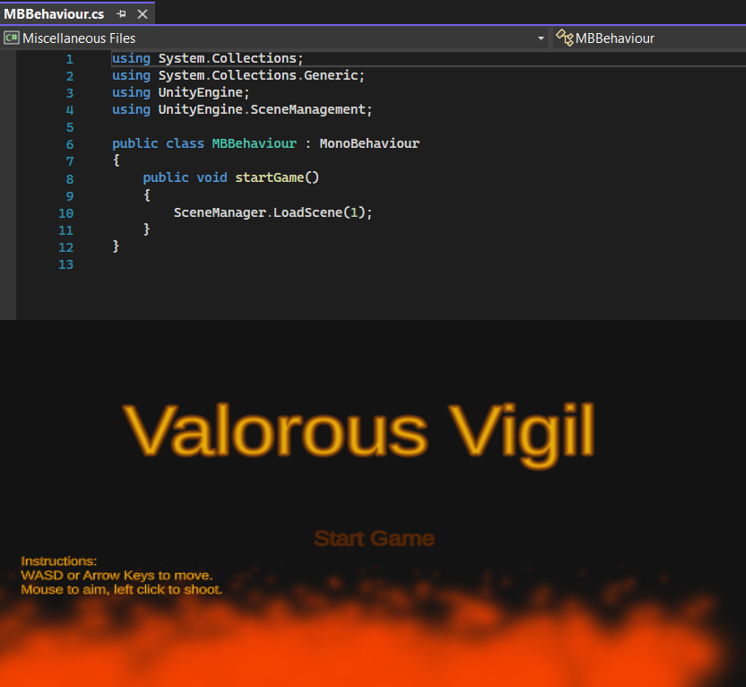
Conclusion
I can safely say this was by far the most challenge prototype so far, with a total of twelve scripts in total required to get it all going smoothly. Using the guidance provided I was able to create something I’m quite proud of whilst at the same time learning various new aspects of Unity that will prove useful in the future such as particle effects. Although there’s no sound in the game it still proves to be a fun little game to play idly and my interest in working further on this project is certainly present.
Additional Content
Using FMOD, I added a soundtrack as well as multiple sound effects to add that extra bit of excitement to each playthrough.
Try out Valorous Vigil here: https://sirdoormat.itch.io/valorous-vigil